Tạo các button điều khiển app nghe nhạc MP3
App nghe nhạc MP3 crawl data từ mp3.zing.vn
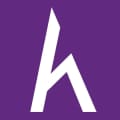
Danh sách bài học
Tạo các button điều khiển app nghe nhạc MP3
Chúng ta cùng nhau tìm hiểu cách để làm ra một app nghe nhạc nhé.
MainStyle.xaml
<ResourceDictionary xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="clr-namespace:MusicAppMP3">
<Style TargetType="TextBlock">
<Setter Property="HorizontalAlignment" Value="Center"></Setter>
<Setter Property="VerticalAlignment" Value="Center"></Setter>
</Style>
</ResourceDictionary>
MainWindow.xaml
<Window x:Class="MusicAppMP3.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:uc="clr-namespace:MusicAppMP3"
xmlns:local="clr-namespace:MusicAppMP3"
mc:Ignorable="d"
WindowStartupLocation="CenterScreen"
FontFamily="Arial"
Title="MainWindow" Height="650" Width="525">
<Window.Resources>
<ResourceDictionary Source="MainStyle.xaml"></ResourceDictionary>
</Window.Resources>
<Grid>
<Grid x:Name="gridTop10">
<Grid.RowDefinitions>
<RowDefinition Height="auto"></RowDefinition>
<RowDefinition></RowDefinition>
</Grid.RowDefinitions>
<Grid>
<Grid.ColumnDefinitions>
<ColumnDefinition></ColumnDefinition>
<ColumnDefinition></ColumnDefinition>
<ColumnDefinition></ColumnDefinition>
</Grid.ColumnDefinitions>
<Grid Grid.Column="0">
<ToggleButton IsChecked="{Binding IsCheckVN}">BXH Việt Nam</ToggleButton>
</Grid>
<Grid Grid.Column="1">
<ToggleButton IsChecked="{Binding IsCheckEU}">BXH Âu Mỹ</ToggleButton>
</Grid>
<Grid Grid.Column="2">
<ToggleButton IsChecked="{Binding IsCheckKO}">BXH Hàn Quốc</ToggleButton>
</Grid>
</Grid>
<Grid Grid.Row="1">
<ListBox x:Name="lsbTopSongs" HorizontalContentAlignment="Stretch">
<ListBox.ItemTemplate>
<DataTemplate>
<Border VerticalAlignment="Stretch" Height="50" BorderThickness="2" BorderBrush="Black">
<Grid>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="50"></ColumnDefinition>
<ColumnDefinition></ColumnDefinition>
<ColumnDefinition Width="50"></ColumnDefinition>
</Grid.ColumnDefinitions>
<Grid>
<TextBlock HorizontalAlignment="Center"
VerticalAlignment="Center">01</TextBlock>
</Grid>
<Grid Grid.Column="1">
<Grid.RowDefinitions>
<RowDefinition></RowDefinition>
<RowDefinition></RowDefinition>
</Grid.RowDefinitions>
<Grid>
<TextBlock HorizontalAlignment="Left" Text="{Binding SongName}"></TextBlock>
</Grid>
<Grid Grid.Row="1">
<TextBlock HorizontalAlignment="Left">Tên ca sỹ</TextBlock>
</Grid>
</Grid>
<Grid Grid.Column="2">
<Button Click="Button_Click">Play</Button>
</Grid>
</Grid>
</Border>
</DataTemplate>
</ListBox.ItemTemplate>
</ListBox>
</Grid>
</Grid>
<uc:SongInfoUC PrevioursClicked="ucSongInfo_PrevioursClicked" NextClicked="ucSongInfo_NextClicked" x:Name="ucSongInfo" Visibility="Hidden"></uc:SongInfoUC>
</Grid>
</Window>
MainWindow.cs
using Newtonsoft.Json.Linq;
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.ComponentModel;
using System.Linq;
using System.Net;
using System.Net.Http;
using System.Text;
using System.Text.RegularExpressions;
using System.Threading.Tasks;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Controls.Primitives;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
using xNet;
namespace MusicAppMP3
{
/// <summary>
/// Interaction logic for MainWindow.xaml
/// </summary>
public partial class MainWindow : Window, INotifyPropertyChanged
{
private bool isCheckVN;
private bool isCheckEU;
private bool isCheckKO;
private ObservableCollection<Song> listVN;
private ObservableCollection<Song> listEU;
private ObservableCollection<Song> listKO;
private Song currentSong;
public bool IsCheckVN { get => isCheckVN; set { isCheckVN = value; lsbTopSongs.ItemsSource = ListVN; isCheckEU = false; isCheckKO = false; OnPropertyChanged("IsCheckVN"); OnPropertyChanged("IsCheckEU"); OnPropertyChanged("IsCheckKO"); } }
public bool IsCheckEU { get => isCheckEU; set { isCheckEU = value; lsbTopSongs.ItemsSource = ListEU; isCheckVN = false; isCheckKO = false; OnPropertyChanged("IsCheckVN"); OnPropertyChanged("IsCheckEU"); OnPropertyChanged("IsCheckKO"); } }
public bool IsCheckKO { get => isCheckKO; set { isCheckKO = value; lsbTopSongs.ItemsSource = ListKO; isCheckEU = false; isCheckVN = false; OnPropertyChanged("IsCheckVN"); OnPropertyChanged("IsCheckEU"); OnPropertyChanged("IsCheckKO"); } }
public ObservableCollection<Song> ListVN { get => listVN; set => listVN = value; }
public ObservableCollection<Song> ListEU { get => listEU; set => listEU = value; }
public ObservableCollection<Song> ListKO { get => listKO; set => listKO = value; }
public Song CurrentSong { get => currentSong; set => currentSong = value; }
public MainWindow()
{
InitializeComponent();
ucSongInfo.BackToMain += UcSongInfo_BackToMain;
this.DataContext = this;
ListVN = new ObservableCollection<Song>();
ListEU = new ObservableCollection<Song>();
ListKO = new ObservableCollection<Song>();
CrawlBXH();
IsCheckVN = true;
}
void CrawlBXH()
{
HttpRequest http = new HttpRequest();
string htmlBXH = http.Get(@"http://mp3.zing.vn/bang-xep-hang/index.html").ToString();
string bxhPattern = @"<div class=""box-chart-ov bordered non-bg-rank"">(.*?)</ul>";
var listBXH = Regex.Matches(htmlBXH, bxhPattern, RegexOptions.Singleline);
string bxhVN = listBXH[0].ToString();
AddSongToListSong(ListVN, bxhVN);
string bxhEU = listBXH[1].ToString();
AddSongToListSong(ListEU, bxhEU);
string bxhKO = listBXH[2].ToString();
AddSongToListSong(ListKO, bxhKO);
}
void AddSongToListSong(ObservableCollection<Song> listSong, string html)
{
var listSongHTML = Regex.Matches(html, @"<li>(.*?)</li>", RegexOptions.Singleline);
for (int i = 0; i < listSongHTML.Count; i++)
{
var songandsinger = Regex.Matches(listSongHTML[i].ToString(), @"<a\s\S*\stitle=""(.*?)""", RegexOptions.Singleline);
string songString = songandsinger[0].ToString();
int indexSong = songString.IndexOf("title=\"");
string songName = songString.Substring(indexSong, songString.Length - indexSong - 1).Replace("title=\"", "");
string singerString = songandsinger[1].ToString();
int indexSinger = singerString.IndexOf("title=\"");
string singerName = singerString.Substring(indexSinger, singerString.Length - indexSinger - 1).Replace("title=\"", "");
int indexURL = songString.IndexOf("href=\"");
string URL = songString.Substring(indexURL, indexSong - indexURL - 2).Replace("href=\"", "");
HttpRequest http = new HttpRequest();
string htmlSong = http.Get(@"http://mp3.zing.vn" + URL).ToString();
var lirycs = Regex.Matches(htmlSong, @"<p class=""fn-wlyrics fn-content""(.*?)</p>", RegexOptions.Singleline);
string tempLiryc = "Chưa có lyric";
if (lirycs.Count > 0)
{
tempLiryc = lirycs[0].ToString();
string tempToCut = tempLiryc.Substring(0, tempLiryc.IndexOf('>') + 1);
tempLiryc = tempLiryc.Replace(tempToCut, "").Replace("<br>", "").Replace("</p>", "");
}
string getJsonURL = Regex.Match(htmlSong, @"<div id=""zplayerjs-wrapper"" class=""player"" data-xml=""(.*?)""", RegexOptions.Singleline).Value.Replace(@"<div id=""zplayerjs-wrapper"" class=""player"" data-xml=""", "").Replace("\"","");
string jsonInfo = http.Get(@"http://mp3.zing.vn" + getJsonURL).ToString();
JObject jObject = JObject.Parse(jsonInfo);
string name = jObject["data"][0]["name"].ToString();
string downloadURL = jObject["data"][0]["source_list"].ToString();
downloadURL = downloadURL.Substring(downloadURL.IndexOf("http"), downloadURL.IndexOf(",") - downloadURL.IndexOf("http") - 1);
string photoURL = jObject["data"][0]["cover"].ToString();
string savePath = AppDomain.CurrentDomain.BaseDirectory + "Song\\" + songName + ".mp3";
listSong.Add(new Song() { SingerName = singerName, SongName = songName, SongURL = URL, STT = i + 1, Lyric=tempLiryc, DownloadURL=downloadURL, PhotoURL = photoURL, SavePath = savePath });
}
}
private void UcSongInfo_BackToMain(object sender, EventArgs e)
{
gridTop10.Visibility = Visibility.Visible;
ucSongInfo.Visibility = Visibility.Hidden;
}
private void Button_Click(object sender, RoutedEventArgs e)
{
Song song = (sender as Button).DataContext as Song;
CurrentSong = song;
gridTop10.Visibility = Visibility.Hidden;
ucSongInfo.Visibility = Visibility.Visible;
ucSongInfo.SongInfo = song;
}
public event PropertyChangedEventHandler PropertyChanged;
protected virtual void OnPropertyChanged(string newName)
{
if (PropertyChanged != null)
{
PropertyChanged(this, new PropertyChangedEventArgs(newName));
}
}
void ChangeToNextWSong(ObservableCollection<Song> listSong, int position, int addCount)
{
int index = listSong.IndexOf(CurrentSong);
if (index == position)
{
return;
}
else
{
CurrentSong = listSong[index + addCount];
ucSongInfo.SongInfo = CurrentSong;
}
}
private void ucSongInfo_PrevioursClicked(object sender, EventArgs e)
{
if (IsCheckVN)
{
ChangeToNextWSong(ListVN, 0, -1);
}
else if (IsCheckEU)
{
ChangeToNextWSong(ListEU, 0, -1);
}
else
{
ChangeToNextWSong(ListKO, 0, -1);
}
}
private void ucSongInfo_NextClicked(object sender, EventArgs e)
{
if (IsCheckVN)
{
ChangeToNextWSong(ListVN, 9, 1);
}
else if (IsCheckEU)
{
ChangeToNextWSong(ListEU, 9, 1);
}
else
{
ChangeToNextWSong(ListKO, 9, 1);
}
}
}
}
SongInfoUC.xaml
<UserControl x:Class="MusicAppMP3.SongInfoUC"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:local="clr-namespace:MusicAppMP3"
mc:Ignorable="d" >
<Grid>
<Grid.RowDefinitions>
<RowDefinition Height="auto"></RowDefinition>
<RowDefinition Height="200"></RowDefinition>
<RowDefinition></RowDefinition>
</Grid.RowDefinitions>
<Grid>
<Button Click="Button_Click">Back</Button>
</Grid>
<Grid Grid.Row="1">
<Grid.RowDefinitions>
<RowDefinition></RowDefinition>
<RowDefinition Height="auto"></RowDefinition>
</Grid.RowDefinitions>
<Canvas x:Name="myCanvas" Grid.Row="0" Background="Orange">
<MediaElement LoadedBehavior="Manual" x:Name="mdAudio" Width="0" Height="0" Source="{Binding SavePath}" MediaOpened="mdAudio_MediaOpened"></MediaElement>
<Grid Width="{Binding ActualWidth, ElementName=myCanvas}"
Height="{Binding ActualHeight, ElementName=myCanvas}">
<Image Source="{Binding PhotoURL}" HorizontalAlignment="Center"
VerticalAlignment="Center" ></Image>
</Grid>
<Grid Width="{Binding ActualWidth, ElementName=myCanvas}"
Height="{Binding ActualHeight, ElementName=myCanvas}">
<Label Content="{Binding SongName}"
HorizontalAlignment="Center"
VerticalAlignment="Center"
/>
</Grid>
</Canvas>
<Grid Grid.Row="1">
<Grid.ColumnDefinitions>
<ColumnDefinition Width="auto"></ColumnDefinition>
<ColumnDefinition></ColumnDefinition>
</Grid.ColumnDefinitions>
<Grid>
<Grid.ColumnDefinitions>
<ColumnDefinition></ColumnDefinition>
<ColumnDefinition></ColumnDefinition>
<ColumnDefinition></ColumnDefinition>
<ColumnDefinition></ColumnDefinition>
</Grid.ColumnDefinitions>
<Grid>
<Button x:Name="btnPlay" Click="Button_Click_2">Play</Button>
</Grid>
<Grid Grid.Column="1">
<Button Click="Button_Click_3">Previours</Button>
</Grid>
<Grid Grid.Column="2">
<Button Click="Button_Click_4">Next</Button>
</Grid>
<Grid Grid.Column="3">
<ToggleButton Checked="ToggleButton_Checked">1.0</ToggleButton>
</Grid>
</Grid>
<Grid Grid.Column="1">
<Grid.ColumnDefinitions>
<ColumnDefinition Width="40"></ColumnDefinition>
<ColumnDefinition></ColumnDefinition>
<ColumnDefinition Width="40"></ColumnDefinition>
</Grid.ColumnDefinitions>
<TextBlock x:Name="txblPosition"></TextBlock>
<Slider Grid.Column="1" PreviewMouseDown="sdDuration_MouseDown" PreviewMouseUp="sdDuration_MouseUp" ValueChanged="sdDuration_ValueChanged" x:Name="sdDuration" HorizontalAlignment="Stretch"></Slider>
<TextBlock x:Name="txblDuration" Grid.Column="2"></TextBlock>
</Grid>
</Grid>
</Grid>
<Grid Grid.Row="2">
<ScrollViewer>
<TextBlock x:Name="txblLiryc" Text="{Binding Lyric}"></TextBlock>
</ScrollViewer>
</Grid>
</Grid>
</UserControl>
SongInfoUC.cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.IO;
using System.Linq;
using System.Net;
using System.Text;
using System.Threading.Tasks;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Controls.Primitives;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
using System.Windows.Threading;
namespace MusicAppMP3
{
/// <summary>
/// Interaction logic for SongInfoUC.xaml
/// </summary>
public partial class SongInfoUC : UserControl, INotifyPropertyChanged
{
private Song songInfo;
public Song SongInfo {
get { return songInfo; }
set
{
songInfo = value;
DownloadSong(SongInfo);
this.DataContext = SongInfo;
OnPropertyChanged("SongInfo");
}
}
private double speedRatio;
public bool IsPlaying { get { return isPlaying; }
set
{
isPlaying = value;
if (isPlaying)
{
mdAudio.Play();
timer.Start();
btnPlay.Content = "Pause";
}
else
{
mdAudio.Pause();
timer.Stop();
btnPlay.Content = "Play";
}
}
}
public double SpeedRatio
{
get { return speedRatio; }
set
{
speedRatio = value;
}
}
private bool isPlaying;
DispatcherTimer timer;
public SongInfoUC()
{
InitializeComponent();
this.DataContext = SongInfo;
timer = new DispatcherTimer();
timer.Interval = new TimeSpan(0, 0, 1);
timer.Tick += Timer_Tick;
SpeedRatio = 1;
}
private void Timer_Tick(object sender, EventArgs e)
{
SongInfo.Position+= SpeedRatio;
sdDuration.Value = SongInfo.Position;
}
private event EventHandler backToMain;
public event EventHandler BackToMain
{
add { backToMain += value; }
remove { backToMain -= value; }
}
void DownloadSong(Song songInfo)
{
string songName = songInfo.SavePath;
if (!File.Exists(songName))
{
WebClient wb = new WebClient();
wb.DownloadFile(SongInfo.DownloadURL, songName);
}
}
private void Button_Click(object sender, RoutedEventArgs e)
{
if (backToMain != null)
backToMain(this, new EventArgs());
}
public event PropertyChangedEventHandler PropertyChanged;
protected virtual void OnPropertyChanged(string newName)
{
if (PropertyChanged != null)
{
PropertyChanged(this, new PropertyChangedEventArgs(newName));
}
}
private void Button_Click_1(object sender, RoutedEventArgs e)
{
}
private void mdAudio_MediaOpened(object sender, RoutedEventArgs e)
{
IsPlaying = true;
SongInfo.Duration = mdAudio.NaturalDuration.TimeSpan.TotalSeconds;
txblDuration.Text = new TimeSpan(0, (int)(SongInfo.Duration / 60), (int)(SongInfo.Duration % 60)).ToString(@"mm\:ss");
sdDuration.Maximum = SongInfo.Duration;
SongInfo.Position = 0;
//timer.Start();
}
bool isDraging = false;
private void sdDuration_ValueChanged(object sender, RoutedPropertyChangedEventArgs<double> e)
{
if (isDraging)
{
SongInfo.Position = sdDuration.Value;
mdAudio.Position = new TimeSpan(0, 0, (int)SongInfo.Position);
}
txblPosition.Text = new TimeSpan(0, (int)(SongInfo.Position / 60), (int)(SongInfo.Position % 60)).ToString(@"mm\:ss");
}
private void sdDuration_MouseDown(object sender, MouseButtonEventArgs e)
{
isDraging = true;
}
private void sdDuration_MouseUp(object sender, MouseButtonEventArgs e)
{
isDraging = false;
}
private void Button_Click_2(object sender, RoutedEventArgs e)
{
IsPlaying = !IsPlaying;
}
private event EventHandler previoursClicked;
public event EventHandler PrevioursClicked
{
add { previoursClicked += value; }
remove { previoursClicked -= value; }
}
private event EventHandler nextClicked;
public event EventHandler NextClicked
{
add { nextClicked += value; }
remove { nextClicked -= value; }
}
private void Button_Click_3(object sender, RoutedEventArgs e)
{
if (previoursClicked != null)
previoursClicked(this, new EventArgs());
}
private void Button_Click_4(object sender, RoutedEventArgs e)
{
if (nextClicked != null)
nextClicked(this, new EventArgs());
}
private void ToggleButton_Checked(object sender, RoutedEventArgs e)
{
ToggleButton toggle = sender as ToggleButton;
if (toggle.IsChecked == true)
{
SpeedRatio = 2;
}
else
{
SpeedRatio = 1;
}
mdAudio.SpeedRatio = SpeedRatio;
toggle.Content = string.Format("{0}.0",SpeedRatio);
}
}
}
Song.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace MusicAppMP3
{
public class Song
{
private string songName;
private string singerName;
private string songURL;
private int sTT;
private string lyric;
private string downloadURL;
private string photoURL;
private string savePath;
private double duration;
private double position;
public string SongName { get => songName; set => songName = value; }
public string SingerName { get => singerName; set => singerName = value; }
public string SongURL { get => songURL; set => songURL = value; }
public int STT { get => sTT; set => sTT = value; }
public string Lyric { get => lyric; set => lyric = value; }
public string DownloadURL { get => downloadURL; set => downloadURL = value; }
public string PhotoURL { get => photoURL; set => photoURL = value; }
public string SavePath { get => savePath; set => savePath = value; }
public double Duration { get => duration; set => duration = value; }
public double Position { get => position; set => position = value; }
}
}
Tải xuống
Tài liệu
Nhằm phục vụ mục đích học tập Offline của cộng đồng, Kteam hỗ trợ tính năng lưu trữ nội dung bài học Tạo các button điều khiển app nghe nhạc MP3 dưới dạng file PDF trong link bên dưới.
Ngoài ra, bạn cũng có thể tìm thấy các tài liệu được đóng góp từ cộng đồng ở mục TÀI LIỆU trên thư viện Howkteam.com
Đừng quên like và share để ủng hộ Kteam và tác giả nhé!
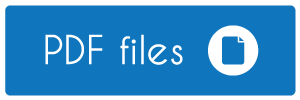
Thảo luận
Nếu bạn có bất kỳ khó khăn hay thắc mắc gì về khóa học, đừng ngần ngại đặt câu hỏi trong phần bên dưới hoặc trong mục HỎI & ĐÁP trên thư viện Howkteam.com để nhận được sự hỗ trợ từ cộng đồng.
Nội dung bài viết
Tác giả/Dịch giả
Khóa học
App nghe nhạc MP3 crawl data từ mp3.zing.vn
App nghe nhạc MP3 crawl data từ mp3.zing.vn
Mong a làm thêm app Android java hoặc Kotlin về chủ đề app nhạc này ạ.
không có Project à anh
mong cái bạn vote cho mình được 100đ với cần bài viết này lắm đề làm đồ án mà kiếm 100đ khó quá huhu
mong cái bạn vote cho mình được 100đ với cần bài viết này lắm đề làm đồ án mà kiếm 100đ khó quá huhu
Bài sau đâu rồi anh.. =))))