Build đa nền tảng Doge game với Unity3D
Lập trình Doge game với Unity3D
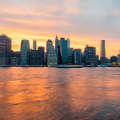
Danh sách bài học
Build đa nền tảng Doge game với Unity3D
Không có gì tuyệt vời hơn là luyện tập với ví dụ thực tế. Nào cùng nhau thử thách bản thân với trò chơi thú vị: Doge game.
Bạn nên có kiến thức về:
- Lập trình C# cơ bản
- Class
- OOP trong C#
File SheepController.cs
using UnityEngine;
using System.Collections;
public class SheepController : MonoBehaviour {
Vector3 mousePos;
public float moveSpeed = 5;
public float minX = -5.5f;
public float maxX = 5.5f;
public float minY = -3;
public float maxY = 3;
private GameObject gameController;
// Use this for initialization
void Start () {
mousePos = transform.position;
gameController = GameObject.FindGameObjectWithTag("GameController");
}
// Update is called once per frame
void Update () {
if (Input.GetMouseButton(0))
{
mousePos = Camera.main.ScreenToWorldPoint(Input.mousePosition);
mousePos = new Vector3(Mathf.Clamp(mousePos.x, minX, maxX), Mathf.Clamp(mousePos.y, minY, maxY), 0);
}
transform.position = Vector3.Lerp(transform.position, mousePos, moveSpeed * Time.deltaTime);
}
void OnTriggerEnter2D(Collider2D other)
{
Destroy(other.gameObject);
gameController.GetComponent<GameController>().EndGame();
}
}
File WolfController.cs
using UnityEngine;
using System.Collections;
public class WolfController : MonoBehaviour {
public GameObject boom;
public float minBoomTime = 2;
public float maxBoomTime = 4;
private float boomTime = 0;
private float lastBoomTime = 0;
public float throughBoomTime = 0.5f;
private GameObject Sheep;
private Animator anim;
private GameObject gameController;
// Use this for initialization
void Start () {
UpdateBoomTime();
Sheep = GameObject.FindGameObjectWithTag("Player");
anim = gameObject.GetComponent<Animator>();
anim.SetBool("isBoom", false);
gameController = GameObject.FindGameObjectWithTag("GameController");
}
// Update is called once per frame
void Update () {
if (Time.time >= lastBoomTime + boomTime - throughBoomTime)
{
anim.SetBool("isBoom", true);
}
if (Time.time >= lastBoomTime + boomTime)
{
ThroughBoom();
}
}
void UpdateBoomTime()
{
lastBoomTime = Time.time;
boomTime = Random.Range(minBoomTime, maxBoomTime + 1);
}
void ThroughBoom()
{
GameObject bom = Instantiate(boom, transform.position, Quaternion.identity) as GameObject;
bom.GetComponent<BoomController>().target = Sheep.transform.position;
UpdateBoomTime();
anim.SetBool("isBoom", false);
gameController.GetComponent<GameController>().GetPoint();
}
}
File BoomController.cs
using UnityEngine;
using System.Collections;
public class BoomController : MonoBehaviour {
public Vector3 target;
public float moveSpeed = 5;
public float destroyTime = 2;
public GameObject explor;
// Use this for initialization
void Start () {
Destroy(gameObject, destroyTime);
}
// Update is called once per frame
void Update () {
transform.Translate((transform.position - target) * moveSpeed * Time.deltaTime * -1);
}
void OnDestroy()
{
GameObject exp = Instantiate(explor, transform.position, Quaternion.identity) as GameObject;
Destroy(exp, 0.5f);
}
}
File GameController.cs
using UnityEngine;
using System.Collections;
using UnityEngine.UI;
using UnityEngine.SceneManagement;
public class GameController : MonoBehaviour {
public GameObject pnlEndGame;
public Button btnRestart;
public Sprite btnIdle;
public Sprite btnHover;
public Sprite btnClick;
public Text txtPoint;
private int gamePoint;
AudioSource audio;
// Use this for initialization
void Start () {
Time.timeScale = 1;
pnlEndGame.SetActive(false);
audio = gameObject.GetComponent<AudioSource>();
}
// Update is called once per frame
void Update () {
}
public void GetPoint()
{
gamePoint++;
txtPoint.text = "Point: " + gamePoint.ToString();
}
public void ButtonHover()
{
btnRestart.GetComponent<Image>().sprite = btnHover;
}
public void ButtonIdle()
{
btnRestart.GetComponent<Image>().sprite = btnIdle;
}
public void ButtonClick()
{
btnRestart.GetComponent<Image>().sprite = btnClick;
}
public void StartGame()
{
SceneManager.LoadScene(0);
}
public void EndGame()
{
audio.Play();
Time.timeScale = 0;
pnlEndGame.SetActive(true);
}
}
File game Demo
Hãy khoe thành quả của bạn với mọi người trong phần bình luận nhé.
Đừng quên: “Luyện tập – Thử thách – Không ngại khó”
Tải xuống
Tài liệu
Nhằm phục vụ mục đích học tập Offline của cộng đồng, Kteam hỗ trợ tính năng lưu trữ nội dung bài học Build đa nền tảng Doge game với Unity3D dưới dạng file PDF trong link bên dưới.
Ngoài ra, bạn cũng có thể tìm thấy các tài liệu được đóng góp từ cộng đồng ở mục TÀI LIỆU trên thư viện Howkteam.com
Đừng quên like và share để ủng hộ Kteam và tác giả nhé!
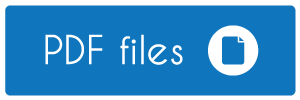
Thảo luận
Nếu bạn có bất kỳ khó khăn hay thắc mắc gì về khóa học, đừng ngần ngại đặt câu hỏi trong phần bên dưới hoặc trong mục HỎI & ĐÁP trên thư viện Howkteam.com để nhận được sự hỗ trợ từ cộng đồng.
Nội dung bài viết
Tác giả/Dịch giả
Khóa học
Lập trình Doge game với Unity3D
Lập trình Doge game với Unity3D
Đánh giá
Hy vọng những bài sau ad sẽ nói chậm và chi tiết hơn.Mình phải tua đi tua lại rất nhiều lần nhưng cũng có những dòng code không hiểu được.Ủng hộ Kteam ra thêm nhiều bài học giá trị nhé.Thanks Kteam rất nhiều.
Hôm nào anh chỉ cái vụ build game rồi up lên Google Play/App Store được không anh? Cảm ơn anh
Đã học và viết xong DogeGame trong 20 giờ.hiểu được 70%,20% mơ hồ ,10% chấp nhận.Thanks Kteam.