Mã hóa mật khẩu trong phần mềm Quản lý quán cafe với C# Winform
Lập trình phần mềm Quản lý quán cafe với C# Winform
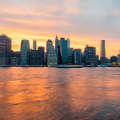
Danh sách bài học
Mã hóa mật khẩu trong phần mềm Quản lý quán cafe với C# Winform
Không có gì tuyệt vời hơn là luyện tập với ví dụ thực tế. Nào cùng nhau thử thách bản thân với phần mềm: Quản lý quán cafe
Bạn nên có kiến thức về:
- Lập trình Winform cơ bản
- Delegate – Event
- SQL server
- Xử lý ngày tháng năm
Code AccountDAO.cs
using QuanLyQuanCafe.DTO;
using System;
using System.Collections.Generic;
using System.Data;
using System.Linq;
using System.Security.Cryptography;
using System.Text;
using System.Threading.Tasks;
namespace QuanLyQuanCafe.DAO
{
public class AccountDAO
{
private static AccountDAO instance;
public static AccountDAO Instance
{
get { if (instance == null) instance = new AccountDAO(); return instance; }
private set { instance = value; }
}
private AccountDAO() { }
public bool Login(string userName, string passWord)
{
byte[] temp = ASCIIEncoding.ASCII.GetBytes(passWord);
byte[] hasData = new MD5CryptoServiceProvider().ComputeHash(temp);
string hasPass = "";
foreach (byte item in hasData)
{
hasPass += item;
}
//var list = hasData.ToString();
//list.Reverse();
string query = "USP_Login @userName , @passWord";
DataTable result = DataProvider.Instance.ExecuteQuery(query, new object[] { userName, hasPass /*list*/});
return result.Rows.Count > 0;
}
public bool UpdateAccount(string userName, string displayName, string pass, string newPass)
{
int result = DataProvider.Instance.ExecuteNonQuery("exec USP_UpdateAccount @userName , @displayName , @password , @newPassword", new object[]{userName, displayName, pass, newPass});
return result > 0;
}
public DataTable GetListAccount()
{
return DataProvider.Instance.ExecuteQuery("SELECT UserName, DisplayName, Type FROM dbo.Account");
}
public Account GetAccountByUserName(string userName)
{
DataTable data = DataProvider.Instance.ExecuteQuery("Select * from account where userName = '" + userName + "'");
foreach (DataRow item in data.Rows)
{
return new Account(item);
}
return null;
}
public bool InsertAccount(string name, string displayName, int type)
{
string query = string.Format("INSERT dbo.Account ( UserName, DisplayName, Type, password )VALUES ( N'{0}', N'{1}', {2}, N'{3}')", name, displayName, type, "1962026656160185351301320480154111117132155");
int result = DataProvider.Instance.ExecuteNonQuery(query);
return result > 0;
}
public bool UpdateAccount(string name, string displayName, int type)
{
string query = string.Format("UPDATE dbo.Account SET DisplayName = N'{1}', Type = {2} WHERE UserName = N'{0}'", name, displayName, type);
int result = DataProvider.Instance.ExecuteNonQuery(query);
return result > 0;
}
public bool DeleteAccount(string name)
{
string query = string.Format("Delete Account where UserName = N'{0}'", name);
int result = DataProvider.Instance.ExecuteNonQuery(query);
return result > 0;
}
public bool ResetPassword(string name)
{
string query = string.Format("update account set password = N'1962026656160185351301320480154111117132155' where UserName = N'{0}'", name);
int result = DataProvider.Instance.ExecuteNonQuery(query);
return result > 0;
}
}
}
Bài sau chúng ta sẽ cùng nhau tìm hiểu cách tạo phím tắt.
Đừng quên: “Luyện tập – Thử thách – Không ngại khó”
Tải xuống
Tài liệu
Nhằm phục vụ mục đích học tập Offline của cộng đồng, Kteam hỗ trợ tính năng lưu trữ nội dung bài học Mã hóa mật khẩu trong phần mềm Quản lý quán cafe với C# Winform dưới dạng file PDF trong link bên dưới.
Ngoài ra, bạn cũng có thể tìm thấy các tài liệu được đóng góp từ cộng đồng ở mục TÀI LIỆU trên thư viện Howkteam.com
Đừng quên like và share để ủng hộ Kteam và tác giả nhé!
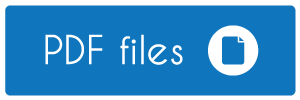
Thảo luận
Nếu bạn có bất kỳ khó khăn hay thắc mắc gì về khóa học, đừng ngần ngại đặt câu hỏi trong phần bên dưới hoặc trong mục HỎI & ĐÁP trên thư viện Howkteam.com để nhận được sự hỗ trợ từ cộng đồng.
Nội dung bài viết
Tác giả/Dịch giả
Khóa học
Lập trình phần mềm Quản lý quán cafe với C# Winform
Bạn đã học qua LẬP TRÌNH C# CƠ BẢN? Xong nốt cả LẬP TRÌNH WINFORM lẫn SQL?
Bạn đã chán các bài tập căn bản, muốn thực hành các kiến thức đã học vào một dự án thực tế?
Hay đơn giản bạn là chủ quán café, mong muốn tự tạo nên phần mềm dành cho chính mình sử dụng?
Vậy còn chần chừ gì không tham gia ngay khóa học LẬP TRÌNH PHẦN MỀM QUÁN CAFÉ VỚI C# WINFORM?
mã hóa như vậy chắc hẳn là theo 1 tập rules cố định. ai cũng xài nó thì nó thành đại trà phải ko nhỉ? người biết được quy luật này chắc phá được phải ko nhỉ?
tạo PROC SQL:
CREATE PROCEDURE [dbo].[sp_AddAccount]
@userName NVARCHAR(100),
@displayName NVARCHAR(100),
@typeAccount INT
AS
BEGIN
DECLARE @exitUserName NVARCHAR(100);
DECLARE @passwordAccount NVARCHAR(100) = N'20720532132149213101239102231223249249135100218';
SELECT @exitUserName = userName FROM dbo.Account WHERE userName = @userName
--PRINT @exitUserName;
IF @exitUserName IS NOT NULL -- Nếu tài khoản tồn tại
BEGIN
UPDATE dbo.Account SET displayName = @displayName, passwordAccount = @passwordAccount, typeAccount = @typeAccount WHERE userName = @userName
PRINT N'Update thành công !';
END
ELSE IF @exitUserName IS NULL
BEGIN
INSERT dbo.Account (userName, displayName, passwordAccount, typeAccount) VALUES (@userName, @displayName, @passwordAccount, @typeAccount)
PRINT N'Insert thành công !';
END
END
GO
Cho em hỏi là cái mã hóa dãy số ý a
Khi insert nó báo lỗi quá 38 kí tự nên k add đc ạ
Cách khắc phục là gì vậy ạ
Khi Mã hoá mật khẩu rồi thì Sửa đổi thông tin lại không có hiệu lực và mật khẩu phải nhập để đổi mật khẩu là đoạn mã hoá ạ. Cách khắc phục ạ.!