Thêm bớt món hóa đơn trong phần mềm Quản lý quán cafe với C# Winform
Lập trình phần mềm Quản lý quán cafe với C# Winform
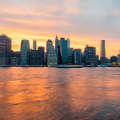
Danh sách bài học
Thêm bớt món hóa đơn trong phần mềm Quản lý quán cafe với C# Winform
Không có gì tuyệt vời hơn là luyện tập với ví dụ thực tế. Nào cùng nhau thử thách bản thân với phần mềm: Quản lý quán cafe
Bạn nên có kiến thức về:
- Lập trình Winform cơ bản
- Delegate – Event
- SQL server
- Xử lý ngày tháng năm
Code fTableManager.cs
using QuanLyQuanCafe.DAO;
using QuanLyQuanCafe.DTO;
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Globalization;
using System.Linq;
using System.Text;
using System.Threading;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace QuanLyQuanCafe
{
public partial class fTableManager : Form
{
public fTableManager()
{
InitializeComponent();
LoadTable();
LoadCategory();
}
#region Method
void LoadCategory()
{
List<Category> listCategory = CategoryDAO.Instance.GetListCategory();
cbCategory.DataSource = listCategory;
cbCategory.DisplayMember = "Name";
}
void LoadFoodListByCategoryID(int id)
{
List<Food> listFood = FoodDAO.Instance.GetFoodByCategoryID(id);
cbFood.DataSource = listFood;
cbFood.DisplayMember = "Name";
}
void LoadTable()
{
List<Table> tableList = TableDAO.Instance.LoadTableList();
foreach (Table item in tableList)
{
Button btn = new Button() { Width = TableDAO.TableWidth, Height = TableDAO.TableHeight};
btn.Text = item.Name + Environment.NewLine + item.Status;
btn.Click += btn_Click;
btn.Tag = item;
switch (item.Status)
{
case "Trống":
btn.BackColor = Color.Aqua;
break;
default:
btn.BackColor = Color.LightPink;
break;
}
flpTable.Controls.Add(btn);
}
}
void ShowBill(int id)
{
lsvBill.Items.Clear();
List<QuanLyQuanCafe.DTO.Menu> listBillInfo = MenuDAO.Instance.GetListMenuByTable(id);
float totalPrice = 0;
foreach (QuanLyQuanCafe.DTO.Menu item in listBillInfo)
{
ListViewItem lsvItem = new ListViewItem(item.FoodName.ToString());
lsvItem.SubItems.Add(item.Count.ToString());
lsvItem.SubItems.Add(item.Price.ToString());
lsvItem.SubItems.Add(item.TotalPrice.ToString());
totalPrice += item.TotalPrice;
lsvBill.Items.Add(lsvItem);
}
CultureInfo culture = new CultureInfo("vi-VN");
//Thread.CurrentThread.CurrentCulture = culture;
txbTotalPrice.Text = totalPrice.ToString("c", culture);
}
#endregion
#region Events
void btn_Click(object sender, EventArgs e)
{
int tableID = ((sender as Button).Tag as Table).ID;
lsvBill.Tag = (sender as Button).Tag;
ShowBill(tableID);
}
private void đăngXuấtToolStripMenuItem_Click(object sender, EventArgs e)
{
this.Close();
}
private void thôngTinCáNhânToolStripMenuItem_Click(object sender, EventArgs e)
{
fAccountProfile f = new fAccountProfile();
f.ShowDialog();
}
private void adminToolStripMenuItem_Click(object sender, EventArgs e)
{
fAdmin f = new fAdmin();
f.ShowDialog();
}
private void cbCategory_SelectedIndexChanged(object sender, EventArgs e)
{
int id = 0;
ComboBox cb = sender as ComboBox;
if (cb.SelectedItem == null)
return;
Category selected = cb.SelectedItem as Category;
id = selected.ID;
LoadFoodListByCategoryID(id);
}
private void btnAddFood_Click(object sender, EventArgs e)
{
Table table = lsvBill.Tag as Table;
int idBill = BillDAO.Instance.GetUncheckBillIDByTableID(table.ID);
int foodID = (cbFood.SelectedItem as Food).ID;
int count = (int)nmFoodCount.Value;
if (idBill == -1)
{
BillDAO.Instance.InsertBill(table.ID);
BillInfoDAO.Instance.InsertBillInfo(BillDAO.Instance.GetMaxIDBill(), foodID , count);
}
else
{
BillInfoDAO.Instance.InsertBillInfo(idBill, foodID, count);
}
ShowBill(table.ID);
}
#endregion
}
}
Code fTableManager.Designer.cs
namespace QuanLyQuanCafe
{
partial class fTableManager
{
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.IContainer components = null;
/// <summary>
/// Clean up any resources being used.
/// </summary>
/// <param name="disposing">true if managed resources should be disposed; otherwise, false.</param>
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.menuStrip1 = new System.Windows.Forms.MenuStrip();
this.adminToolStripMenuItem = new System.Windows.Forms.ToolStripMenuItem();
this.thôngTinTàiKhoảnToolStripMenuItem = new System.Windows.Forms.ToolStripMenuItem();
this.thôngTinCáNhânToolStripMenuItem = new System.Windows.Forms.ToolStripMenuItem();
this.đăngXuấtToolStripMenuItem = new System.Windows.Forms.ToolStripMenuItem();
this.panel2 = new System.Windows.Forms.Panel();
this.lsvBill = new System.Windows.Forms.ListView();
this.columnHeader1 = ((System.Windows.Forms.ColumnHeader)(new System.Windows.Forms.ColumnHeader()));
this.columnHeader2 = ((System.Windows.Forms.ColumnHeader)(new System.Windows.Forms.ColumnHeader()));
this.columnHeader3 = ((System.Windows.Forms.ColumnHeader)(new System.Windows.Forms.ColumnHeader()));
this.columnHeader4 = ((System.Windows.Forms.ColumnHeader)(new System.Windows.Forms.ColumnHeader()));
this.panel3 = new System.Windows.Forms.Panel();
this.txbTotalPrice = new System.Windows.Forms.TextBox();
this.cbSwitchTable = new System.Windows.Forms.ComboBox();
this.btnSwitchTable = new System.Windows.Forms.Button();
this.nmDisCount = new System.Windows.Forms.NumericUpDown();
this.btnDiscount = new System.Windows.Forms.Button();
this.btnCheckOut = new System.Windows.Forms.Button();
this.panel4 = new System.Windows.Forms.Panel();
this.nmFoodCount = new System.Windows.Forms.NumericUpDown();
this.btnAddFood = new System.Windows.Forms.Button();
this.cbFood = new System.Windows.Forms.ComboBox();
this.cbCategory = new System.Windows.Forms.ComboBox();
this.flpTable = new System.Windows.Forms.FlowLayoutPanel();
this.menuStrip1.SuspendLayout();
this.panel2.SuspendLayout();
this.panel3.SuspendLayout();
((System.ComponentModel.ISupportInitialize)(this.nmDisCount)).BeginInit();
this.panel4.SuspendLayout();
((System.ComponentModel.ISupportInitialize)(this.nmFoodCount)).BeginInit();
this.SuspendLayout();
//
// menuStrip1
//
this.menuStrip1.Items.AddRange(new System.Windows.Forms.ToolStripItem[] {
this.adminToolStripMenuItem,
this.thôngTinTàiKhoảnToolStripMenuItem});
this.menuStrip1.Location = new System.Drawing.Point(0, 0);
this.menuStrip1.Name = "menuStrip1";
this.menuStrip1.Size = new System.Drawing.Size(797, 24);
this.menuStrip1.TabIndex = 1;
this.menuStrip1.Text = "menuStrip1";
//
// adminToolStripMenuItem
//
this.adminToolStripMenuItem.Name = "adminToolStripMenuItem";
this.adminToolStripMenuItem.Size = new System.Drawing.Size(55, 20);
this.adminToolStripMenuItem.Text = "Admin";
this.adminToolStripMenuItem.Click += new System.EventHandler(this.adminToolStripMenuItem_Click);
//
// thôngTinTàiKhoảnToolStripMenuItem
//
this.thôngTinTàiKhoảnToolStripMenuItem.DropDownItems.AddRange(new System.Windows.Forms.ToolStripItem[] {
this.thôngTinCáNhânToolStripMenuItem,
this.đăngXuấtToolStripMenuItem});
this.thôngTinTàiKhoảnToolStripMenuItem.Name = "thôngTinTàiKhoảnToolStripMenuItem";
this.thôngTinTàiKhoảnToolStripMenuItem.Size = new System.Drawing.Size(123, 20);
this.thôngTinTàiKhoảnToolStripMenuItem.Text = "Thông tin tài khoản";
//
// thôngTinCáNhânToolStripMenuItem
//
this.thôngTinCáNhânToolStripMenuItem.Name = "thôngTinCáNhânToolStripMenuItem";
this.thôngTinCáNhânToolStripMenuItem.Size = new System.Drawing.Size(171, 22);
this.thôngTinCáNhânToolStripMenuItem.Text = "Thông tin cá nhân";
this.thôngTinCáNhânToolStripMenuItem.Click += new System.EventHandler(this.thôngTinCáNhânToolStripMenuItem_Click);
//
// đăngXuấtToolStripMenuItem
//
this.đăngXuấtToolStripMenuItem.Name = "đăngXuấtToolStripMenuItem";
this.đăngXuấtToolStripMenuItem.Size = new System.Drawing.Size(171, 22);
this.đăngXuấtToolStripMenuItem.Text = "Đăng xuất";
this.đăngXuấtToolStripMenuItem.Click += new System.EventHandler(this.đăngXuấtToolStripMenuItem_Click);
//
// panel2
//
this.panel2.Controls.Add(this.lsvBill);
this.panel2.Location = new System.Drawing.Point(447, 85);
this.panel2.Name = "panel2";
this.panel2.Size = new System.Drawing.Size(338, 315);
this.panel2.TabIndex = 2;
//
// lsvBill
//
this.lsvBill.Columns.AddRange(new System.Windows.Forms.ColumnHeader[] {
this.columnHeader1,
this.columnHeader2,
this.columnHeader3,
this.columnHeader4});
this.lsvBill.GridLines = true;
this.lsvBill.Location = new System.Drawing.Point(3, 3);
this.lsvBill.Name = "lsvBill";
this.lsvBill.Size = new System.Drawing.Size(332, 309);
this.lsvBill.TabIndex = 0;
this.lsvBill.UseCompatibleStateImageBehavior = false;
this.lsvBill.View = System.Windows.Forms.View.Details;
//
// columnHeader1
//
this.columnHeader1.Text = "Tên món";
this.columnHeader1.Width = 133;
//
// columnHeader2
//
this.columnHeader2.Text = "Số lượng";
//
// columnHeader3
//
this.columnHeader3.Text = "Đơn giá";
this.columnHeader3.Width = 54;
//
// columnHeader4
//
this.columnHeader4.Text = "Thành tiền";
this.columnHeader4.Width = 81;
//
// panel3
//
this.panel3.Controls.Add(this.txbTotalPrice);
this.panel3.Controls.Add(this.cbSwitchTable);
this.panel3.Controls.Add(this.btnSwitchTable);
this.panel3.Controls.Add(this.nmDisCount);
this.panel3.Controls.Add(this.btnDiscount);
this.panel3.Controls.Add(this.btnCheckOut);
this.panel3.Location = new System.Drawing.Point(447, 406);
this.panel3.Name = "panel3";
this.panel3.Size = new System.Drawing.Size(338, 52);
this.panel3.TabIndex = 3;
//
// txbTotalPrice
//
this.txbTotalPrice.Font = new System.Drawing.Font("Arial", 11.25F, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, ((byte)(163)));
this.txbTotalPrice.ForeColor = System.Drawing.Color.OrangeRed;
this.txbTotalPrice.Location = new System.Drawing.Point(164, 17);
this.txbTotalPrice.Name = "txbTotalPrice";
this.txbTotalPrice.ReadOnly = true;
this.txbTotalPrice.Size = new System.Drawing.Size(90, 25);
this.txbTotalPrice.TabIndex = 7;
this.txbTotalPrice.Text = "0";
this.txbTotalPrice.TextAlign = System.Windows.Forms.HorizontalAlignment.Right;
//
// cbSwitchTable
//
this.cbSwitchTable.FormattingEnabled = true;
this.cbSwitchTable.Location = new System.Drawing.Point(3, 28);
this.cbSwitchTable.Name = "cbSwitchTable";
this.cbSwitchTable.Size = new System.Drawing.Size(75, 21);
this.cbSwitchTable.TabIndex = 6;
//
// btnSwitchTable
//
this.btnSwitchTable.Location = new System.Drawing.Point(3, 3);
this.btnSwitchTable.Name = "btnSwitchTable";
this.btnSwitchTable.Size = new System.Drawing.Size(75, 26);
this.btnSwitchTable.TabIndex = 5;
this.btnSwitchTable.Text = "Chuyển bàn";
this.btnSwitchTable.UseVisualStyleBackColor = true;
//
// nmDisCount
//
this.nmDisCount.Location = new System.Drawing.Point(84, 29);
this.nmDisCount.Name = "nmDisCount";
this.nmDisCount.Size = new System.Drawing.Size(74, 20);
this.nmDisCount.TabIndex = 4;
this.nmDisCount.TextAlign = System.Windows.Forms.HorizontalAlignment.Center;
//
// btnDiscount
//
this.btnDiscount.Location = new System.Drawing.Point(84, 3);
this.btnDiscount.Name = "btnDiscount";
this.btnDiscount.Size = new System.Drawing.Size(75, 26);
this.btnDiscount.TabIndex = 4;
this.btnDiscount.Text = "Giảm giá";
this.btnDiscount.UseVisualStyleBackColor = true;
//
// btnCheckOut
//
this.btnCheckOut.Location = new System.Drawing.Point(260, 3);
this.btnCheckOut.Name = "btnCheckOut";
this.btnCheckOut.Size = new System.Drawing.Size(75, 46);
this.btnCheckOut.TabIndex = 3;
this.btnCheckOut.Text = "Thanh toán";
this.btnCheckOut.UseVisualStyleBackColor = true;
//
// panel4
//
this.panel4.Controls.Add(this.nmFoodCount);
this.panel4.Controls.Add(this.btnAddFood);
this.panel4.Controls.Add(this.cbFood);
this.panel4.Controls.Add(this.cbCategory);
this.panel4.Location = new System.Drawing.Point(447, 27);
this.panel4.Name = "panel4";
this.panel4.Size = new System.Drawing.Size(338, 52);
this.panel4.TabIndex = 4;
//
// nmFoodCount
//
this.nmFoodCount.Location = new System.Drawing.Point(296, 19);
this.nmFoodCount.Minimum = new decimal(new int[] {
100,
0,
0,
-2147483648});
this.nmFoodCount.Name = "nmFoodCount";
this.nmFoodCount.Size = new System.Drawing.Size(39, 20);
this.nmFoodCount.TabIndex = 3;
this.nmFoodCount.Value = new decimal(new int[] {
1,
0,
0,
0});
//
// btnAddFood
//
this.btnAddFood.Location = new System.Drawing.Point(214, 3);
this.btnAddFood.Name = "btnAddFood";
this.btnAddFood.Size = new System.Drawing.Size(75, 46);
this.btnAddFood.TabIndex = 2;
this.btnAddFood.Text = "Thêm món";
this.btnAddFood.UseVisualStyleBackColor = true;
this.btnAddFood.Click += new System.EventHandler(this.btnAddFood_Click);
//
// cbFood
//
this.cbFood.FormattingEnabled = true;
this.cbFood.Location = new System.Drawing.Point(3, 28);
this.cbFood.Name = "cbFood";
this.cbFood.Size = new System.Drawing.Size(205, 21);
this.cbFood.TabIndex = 1;
//
// cbCategory
//
this.cbCategory.FormattingEnabled = true;
this.cbCategory.Location = new System.Drawing.Point(3, 3);
this.cbCategory.Name = "cbCategory";
this.cbCategory.Size = new System.Drawing.Size(205, 21);
this.cbCategory.TabIndex = 0;
this.cbCategory.SelectedIndexChanged += new System.EventHandler(this.cbCategory_SelectedIndexChanged);
//
// flpTable
//
this.flpTable.AutoScroll = true;
this.flpTable.Location = new System.Drawing.Point(12, 30);
this.flpTable.Name = "flpTable";
this.flpTable.Size = new System.Drawing.Size(429, 428);
this.flpTable.TabIndex = 5;
//
// fTableManager
//
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 13F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(797, 470);
this.Controls.Add(this.flpTable);
this.Controls.Add(this.panel4);
this.Controls.Add(this.panel3);
this.Controls.Add(this.panel2);
this.Controls.Add(this.menuStrip1);
this.MainMenuStrip = this.menuStrip1;
this.Name = "fTableManager";
this.StartPosition = System.Windows.Forms.FormStartPosition.CenterScreen;
this.Text = "Phần mềm quản lý quán cafe";
this.menuStrip1.ResumeLayout(false);
this.menuStrip1.PerformLayout();
this.panel2.ResumeLayout(false);
this.panel3.ResumeLayout(false);
this.panel3.PerformLayout();
((System.ComponentModel.ISupportInitialize)(this.nmDisCount)).EndInit();
this.panel4.ResumeLayout(false);
((System.ComponentModel.ISupportInitialize)(this.nmFoodCount)).EndInit();
this.ResumeLayout(false);
this.PerformLayout();
}
#endregion
private System.Windows.Forms.MenuStrip menuStrip1;
private System.Windows.Forms.ToolStripMenuItem adminToolStripMenuItem;
private System.Windows.Forms.ToolStripMenuItem thôngTinTàiKhoảnToolStripMenuItem;
private System.Windows.Forms.ToolStripMenuItem thôngTinCáNhânToolStripMenuItem;
private System.Windows.Forms.ToolStripMenuItem đăngXuấtToolStripMenuItem;
private System.Windows.Forms.Panel panel2;
private System.Windows.Forms.ListView lsvBill;
private System.Windows.Forms.Panel panel3;
private System.Windows.Forms.Panel panel4;
private System.Windows.Forms.NumericUpDown nmFoodCount;
private System.Windows.Forms.Button btnAddFood;
private System.Windows.Forms.ComboBox cbFood;
private System.Windows.Forms.ComboBox cbCategory;
private System.Windows.Forms.ComboBox cbSwitchTable;
private System.Windows.Forms.Button btnSwitchTable;
private System.Windows.Forms.NumericUpDown nmDisCount;
private System.Windows.Forms.Button btnDiscount;
private System.Windows.Forms.Button btnCheckOut;
private System.Windows.Forms.FlowLayoutPanel flpTable;
private System.Windows.Forms.ColumnHeader columnHeader1;
private System.Windows.Forms.ColumnHeader columnHeader2;
private System.Windows.Forms.ColumnHeader columnHeader3;
private System.Windows.Forms.ColumnHeader columnHeader4;
private System.Windows.Forms.TextBox txbTotalPrice;
}
}
Code FoodDAO.cs
using QuanLyQuanCafe.DTO;
using System;
using System.Collections.Generic;
using System.Data;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace QuanLyQuanCafe.DAO
{
public class FoodDAO
{
private static FoodDAO instance;
public static FoodDAO Instance
{
get { if (instance == null)instance = new FoodDAO(); return FoodDAO.instance; }
private set { FoodDAO.instance = value; }
}
private FoodDAO() { }
public List<Food> GetFoodByCategoryID(int id)
{
List<Food> list = new List<Food>();
string query = "select * from Food where idCategory = " + id;
DataTable data = DataProvider.Instance.ExecuteQuery(query);
foreach (DataRow item in data.Rows)
{
Food food = new Food(item);
list.Add(food);
}
return list;
}
}
}
Code Food.cs
using System;
using System.Collections.Generic;
using System.Data;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace QuanLyQuanCafe.DTO
{
public class Food
{
public Food(int id, string name, int categoryID, float price)
{
this.ID = id;
this.Name = name;
this.CategoryID = categoryID;
this.Price = price;
}
public Food(DataRow row)
{
this.ID = (int)row["id"];
this.Name = row["name"].ToString();
this.CategoryID = (int)row["idcategory"];
this.Price = (float)Convert.ToDouble(row["price"].ToString());
}
private float price;
public float Price
{
get { return price; }
set { price = value; }
}
private int categoryID;
public int CategoryID
{
get { return categoryID; }
set { categoryID = value; }
}
private string name;
public string Name
{
get { return name; }
set { name = value; }
}
private int iD;
public int ID
{
get { return iD; }
set { iD = value; }
}
}
}
Code CategoryDAO.cs
using QuanLyQuanCafe.DTO;
using System;
using System.Collections.Generic;
using System.Data;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace QuanLyQuanCafe.DAO
{
public class CategoryDAO
{
private static CategoryDAO instance;
public static CategoryDAO Instance
{
get { if (instance == null)instance = new CategoryDAO(); return CategoryDAO.instance; }
private set { CategoryDAO.instance = value; }
}
private CategoryDAO() { }
public List<Category> GetListCategory()
{
List<Category> list = new List<Category>();
string query = "select * from FoodCategory";
DataTable data = DataProvider.Instance.ExecuteQuery(query);
foreach (DataRow item in data.Rows)
{
Category category = new Category(item);
list.Add(category);
}
return list;
}
}
}
Code Category.cs
using System;
using System.Collections.Generic;
using System.Data;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace QuanLyQuanCafe.DTO
{
public class Category
{
public Category(int id, string name)
{
this.ID = id;
this.Name = name;
}
public Category(DataRow row)
{
this.ID = (int)row["id"];
this.Name = row["name"].ToString();
}
private string name;
public string Name
{
get { return name; }
set { name = value; }
}
private int iD;
public int ID
{
get { return iD; }
set { iD = value; }
}
}
}
Code BillInfoDAO.cs
using QuanLyQuanCafe.DTO;
using System;
using System.Collections.Generic;
using System.Data;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace QuanLyQuanCafe.DAO
{
public class BillInfoDAO
{
private static BillInfoDAO instance;
public static BillInfoDAO Instance
{
get { if (instance == null) instance = new BillInfoDAO(); return BillInfoDAO.instance; }
private set { BillInfoDAO.instance = value; }
}
private BillInfoDAO() { }
public List<BillInfo> GetListBillInfo(int id)
{
List<BillInfo> listBillInfo = new List<BillInfo>();
DataTable data = DataProvider.Instance.ExecuteQuery("SELECT * FROM dbo.BillInfo WHERE idBill = " + id);
foreach (DataRow item in data.Rows)
{
BillInfo info = new BillInfo(item);
listBillInfo.Add(info);
}
return listBillInfo;
}
public void InsertBillInfo(int idBill, int idFood, int count)
{
DataProvider.Instance.ExecuteNonQuery("USP_InsertBillInfo @idBill , @idFood , @count", new object[] { idBill, idFood, count });
}
}
}
Code BillDAO.cs
using QuanLyQuanCafe.DTO;
using System;
using System.Collections.Generic;
using System.Data;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace QuanLyQuanCafe.DAO
{
public class BillDAO
{
private static BillDAO instance;
public static BillDAO Instance
{
get { if (instance == null) instance = new BillDAO(); return BillDAO.instance; }
private set { BillDAO.instance = value; }
}
private BillDAO() { }
/// <summary>
/// Thành công: bill ID
/// thất bại: -1
/// </summary>
/// <param name="id"></param>
/// <returns></returns>
public int GetUncheckBillIDByTableID(int id)
{
DataTable data = DataProvider.Instance.ExecuteQuery("SELECT * FROM dbo.Bill WHERE idTable = " + id +" AND status = 0");
if (data.Rows.Count > 0)
{
Bill bill = new Bill(data.Rows[0]);
return bill.ID;
}
return -1;
}
public void InsertBill(int id)
{
DataProvider.Instance.ExecuteNonQuery("exec USP_InsertBill @idTable", new object[]{id});
}
public int GetMaxIDBill()
{
try
{
return (int)DataProvider.Instance.ExecuteScalar("SELECT MAX(id) FROM dbo.Bill");
}
catch
{
return 1;
}
}
}
}
Bài sau chúng ta sẽ cùng nhau tìm hiểu về thanh toán hóa đơn.
Đừng quên: “Luyện tập – Thử thách – Không ngại khó”
Tải xuống
Tài liệu
Nhằm phục vụ mục đích học tập Offline của cộng đồng, Kteam hỗ trợ tính năng lưu trữ nội dung bài học Thêm bớt món hóa đơn trong phần mềm Quản lý quán cafe với C# Winform dưới dạng file PDF trong link bên dưới.
Ngoài ra, bạn cũng có thể tìm thấy các tài liệu được đóng góp từ cộng đồng ở mục TÀI LIỆU trên thư viện Howkteam.com
Đừng quên like và share để ủng hộ Kteam và tác giả nhé!
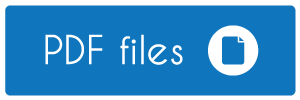
Thảo luận
Nếu bạn có bất kỳ khó khăn hay thắc mắc gì về khóa học, đừng ngần ngại đặt câu hỏi trong phần bên dưới hoặc trong mục HỎI & ĐÁP trên thư viện Howkteam.com để nhận được sự hỗ trợ từ cộng đồng.
Nội dung bài viết
Tác giả/Dịch giả
Khóa học
Lập trình phần mềm Quản lý quán cafe với C# Winform
Bạn đã học qua LẬP TRÌNH C# CƠ BẢN? Xong nốt cả LẬP TRÌNH WINFORM lẫn SQL?
Bạn đã chán các bài tập căn bản, muốn thực hành các kiến thức đã học vào một dự án thực tế?
Hay đơn giản bạn là chủ quán café, mong muốn tự tạo nên phần mềm dành cho chính mình sử dụng?
Vậy còn chần chừ gì không tham gia ngay khóa học LẬP TRÌNH PHẦN MỀM QUÁN CAFÉ VỚI C# WINFORM?
System.Data.SqlClient.SqlException: 'Incorrect syntax near ','.
Must declare the scalar variable "@idBILL".'
em bị lỗi này thì fix như nào v ạ em mò cả ngày nay rồi
thưa anh trong quá trình làm em bị lỗi form sau đó em bấm Ignore and Continue thì nó ra một cái trắng tinh, h ko bt làm cách nào để quay lại form ban đầu đã thiết kế ạ?
var DateCheckOutTemp = (DateTime?)row["dateCheckOut"];
if (DateCheckOutTemp.ToString() != "")
{
this.DateCheckOut = (DateTime?)DateCheckOutTemp;
}
Đoạn trên bị lỗi như này là sao anh : System.InvalidCastException: 'Specified cast is not valid.'
System.Data.SqlClient.SqlException: 'Could not find stored procedure 'USP_InsertBill'.' em bị lỗi chỗ này ad fix giúp em. em cảm ơn ạ
Anh ơi cho em hỏi là :
Khi em thêm món ăn thì những món giống nhau ko update số lượng mà nó cứ thành từ dòng như một món mới, và lúc mà thêm số lượng âm thì cũng hiện trong Bill, chỉ trừ tiền chứ ko bị mất món đó.
Mong anh giúp đỡ