Entity Framework trong lập trình C# Winform
Lập trình Winform cơ bản
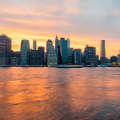
Danh sách bài học
Entity Framework trong lập trình C# Winform
Dẫn nhập
Sức mạnh của hệ điều hành Window là không thể chối cãi. Và để tạo nên sức mạnh đó không thể thiếu những ứng dụng mạnh mẽ. Vậy để tạo ra những ứng dụng đó, người lập trình viên cần học cái gì?
Cùng nhau tìm hiểu serial Lập trình Winform.
Nội dung
SinhVien.cs
//------------------------------------------------------------------------------
// <auto-generated>
// This code was generated from a template.
//
// Manual changes to this file may cause unexpected behavior in your application.
// Manual changes to this file will be overwritten if the code is regenerated.
// </auto-generated>
//------------------------------------------------------------------------------
namespace EntityGUI
{
using System;
using System.Collections.Generic;
public partial class SinhVien
{
public int ID { get; set; }
public string Name { get; set; }
public int IDLop { get; set; }
public virtual Lop Lop { get; set; }
}
}
Lop.cs
//------------------------------------------------------------------------------
// <auto-generated>
// This code was generated from a template.
//
// Manual changes to this file may cause unexpected behavior in your application.
// Manual changes to this file will be overwritten if the code is regenerated.
// </auto-generated>
//------------------------------------------------------------------------------
namespace EntityGUI
{
using System;
using System.Collections.Generic;
public partial class Lop
{
[System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA2214:DoNotCallOverridableMethodsInConstructors")]
public Lop()
{
this.SinhVien = new HashSet<SinhVien>();
}
public int ID { get; set; }
public string Name { get; set; }
[System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA2227:CollectionPropertiesShouldBeReadOnly")]
public virtual ICollection<SinhVien> SinhVien { get; set; }
}
}
Form1.cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace EntityGUI
{
public partial class Form1 : Form
{
KteamEntities db = new KteamEntities();
public Form1()
{
InitializeComponent();
LoadData();
AddBinding();
}
#region methods
void AddBinding()
{
txbID.DataBindings.Add(new Binding("Text", dtgvData.DataSource, "IDLop", true, DataSourceUpdateMode.Never));
txbName.DataBindings.Add(new Binding("Text", dtgvData.DataSource, "Name", true, DataSourceUpdateMode.Never));
}
void LoadData()
{
//using (KteamEntities db = new KteamEntities())
{
var result = from c in db.SinhVien
//where c.ID > 1 && c.ID < 4
select c;
//var result = db.SinhVien.Find(2);
dtgvData.DataSource = result.ToList();
}
}
void AddSinhVien()
{
//using (KteamEntities db = new KteamEntities())
{
SinhVien sv = new SinhVien() { Name = txbName.Text, IDLop = Convert.ToInt32(txbID.Text)};
db.SinhVien.Add(sv);
db.SaveChanges();
}
}
void DeleteSinhVien()
{
int id = Convert.ToInt32(txbID.Text);
SinhVien sv = db.SinhVien.Where(p => p.IDLop == id && p.Name == txbName.Text).SingleOrDefault();
db.SinhVien.Remove(sv);
db.SaveChanges();
}
void EditSinhVien()
{
int id = Convert.ToInt32(dtgvData.SelectedCells[0].OwningRow.Cells["ID"].Value.ToString());
SinhVien sv = db.SinhVien.Find(id);
sv.Name = txbName.Text;
sv.IDLop = Convert.ToInt32(txbID.Text);
db.SaveChanges();
}
#endregion
#region Events
private void btnShow_Click(object sender, EventArgs e)
{
LoadData();
}
private void btnAdd_Click(object sender, EventArgs e)
{
AddSinhVien();
}
private void btnDelete_Click(object sender, EventArgs e)
{
DeleteSinhVien();
}
private void btnEdit_Click(object sender, EventArgs e)
{
EditSinhVien();
}
#endregion
}
}
Form1.Designer.cs
namespace EntityGUI
{
partial class Form1
{
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.IContainer components = null;
/// <summary>
/// Clean up any resources being used.
/// </summary>
/// <param name="disposing">true if managed resources should be disposed; otherwise, false.</param>
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.dtgvData = new System.Windows.Forms.DataGridView();
this.txbID = new System.Windows.Forms.TextBox();
this.txbName = new System.Windows.Forms.TextBox();
this.btnShow = new System.Windows.Forms.Button();
this.btnAdd = new System.Windows.Forms.Button();
this.btnDelete = new System.Windows.Forms.Button();
this.btnEdit = new System.Windows.Forms.Button();
((System.ComponentModel.ISupportInitialize)(this.dtgvData)).BeginInit();
this.SuspendLayout();
//
// dtgvData
//
this.dtgvData.ColumnHeadersHeightSizeMode = System.Windows.Forms.DataGridViewColumnHeadersHeightSizeMode.AutoSize;
this.dtgvData.Location = new System.Drawing.Point(12, 40);
this.dtgvData.Name = "dtgvData";
this.dtgvData.Size = new System.Drawing.Size(239, 323);
this.dtgvData.TabIndex = 0;
//
// txbID
//
this.txbID.Location = new System.Drawing.Point(257, 40);
this.txbID.Name = "txbID";
this.txbID.Size = new System.Drawing.Size(100, 20);
this.txbID.TabIndex = 1;
//
// txbName
//
this.txbName.Location = new System.Drawing.Point(257, 77);
this.txbName.Name = "txbName";
this.txbName.Size = new System.Drawing.Size(100, 20);
this.txbName.TabIndex = 2;
//
// btnShow
//
this.btnShow.Location = new System.Drawing.Point(257, 103);
this.btnShow.Name = "btnShow";
this.btnShow.Size = new System.Drawing.Size(75, 23);
this.btnShow.TabIndex = 3;
this.btnShow.Text = "Xem";
this.btnShow.UseVisualStyleBackColor = true;
this.btnShow.Click += new System.EventHandler(this.btnShow_Click);
//
// btnAdd
//
this.btnAdd.Location = new System.Drawing.Point(257, 132);
this.btnAdd.Name = "btnAdd";
this.btnAdd.Size = new System.Drawing.Size(75, 23);
this.btnAdd.TabIndex = 4;
this.btnAdd.Text = "Thêm";
this.btnAdd.UseVisualStyleBackColor = true;
this.btnAdd.Click += new System.EventHandler(this.btnAdd_Click);
//
// btnDelete
//
this.btnDelete.Location = new System.Drawing.Point(257, 161);
this.btnDelete.Name = "btnDelete";
this.btnDelete.Size = new System.Drawing.Size(75, 23);
this.btnDelete.TabIndex = 5;
this.btnDelete.Text = "Xóa";
this.btnDelete.UseVisualStyleBackColor = true;
this.btnDelete.Click += new System.EventHandler(this.btnDelete_Click);
//
// btnEdit
//
this.btnEdit.Location = new System.Drawing.Point(257, 190);
this.btnEdit.Name = "btnEdit";
this.btnEdit.Size = new System.Drawing.Size(75, 23);
this.btnEdit.TabIndex = 6;
this.btnEdit.Text = "Sửa";
this.btnEdit.UseVisualStyleBackColor = true;
this.btnEdit.Click += new System.EventHandler(this.btnEdit_Click);
//
// Form1
//
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 13F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(531, 375);
this.Controls.Add(this.btnEdit);
this.Controls.Add(this.btnDelete);
this.Controls.Add(this.btnAdd);
this.Controls.Add(this.btnShow);
this.Controls.Add(this.txbName);
this.Controls.Add(this.txbID);
this.Controls.Add(this.dtgvData);
this.Name = "Form1";
this.Text = "Form1";
((System.ComponentModel.ISupportInitialize)(this.dtgvData)).EndInit();
this.ResumeLayout(false);
this.PerformLayout();
}
#endregion
private System.Windows.Forms.DataGridView dtgvData;
private System.Windows.Forms.TextBox txbID;
private System.Windows.Forms.TextBox txbName;
private System.Windows.Forms.Button btnShow;
private System.Windows.Forms.Button btnAdd;
private System.Windows.Forms.Button btnDelete;
private System.Windows.Forms.Button btnEdit;
}
}
Kết luận
Tham khảo thêm nhiều khóa học Lập trình C#.net thực tế khác tại Howkteam.com
Cảm ơn các bạn đã theo dõi bài viết. Hãy để lại bình luận hoặc góp ý của mình để phát triển bài viết tốt hơn. Đừng quên “Luyện tập – Thử thách – Không ngại khó”.
Tải xuống
Tài liệu
Nhằm phục vụ mục đích học tập Offline của cộng đồng, Kteam hỗ trợ tính năng lưu trữ nội dung bài học Entity Framework trong lập trình C# Winform dưới dạng file PDF trong link bên dưới.
Ngoài ra, bạn cũng có thể tìm thấy các tài liệu được đóng góp từ cộng đồng ở mục TÀI LIỆU trên thư viện Howkteam.com
Đừng quên like và share để ủng hộ Kteam và tác giả nhé!
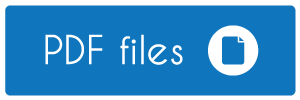
Thảo luận
Nếu bạn có bất kỳ khó khăn hay thắc mắc gì về khóa học, đừng ngần ngại đặt câu hỏi trong phần bên dưới hoặc trong mục HỎI & ĐÁP trên thư viện Howkteam.com để nhận được sự hỗ trợ từ cộng đồng.
Nội dung bài viết
Tác giả/Dịch giả
Khóa học
Lập trình Winform cơ bản
Dạ có thể giúp e làm sao để thể hiện model trên EF đc ko ạ ? e ko biết thể hiện images (string array) này thế nào
{
"id": 13303321,
"code": "SP032388",
"inventories": [
{
"productId": 13303321,
"onHand": 0,
"reserved": 0,
},
{
"productId": 13303321,
"onHand": 0,
"reserved": 5
}
],
"images": [
"https://cdn-images.kiotviet.vn/0798008216/314ead935e53402a87791de0fee729c7.jpeg",
"https://cdn-images.kiotviet.vn/0798008216/f0a2980ea9fa4c8493de7387764001af.jpeg",
"https://cdn-images.kiotviet.vn/0798008216/43b58977595a43c08416ddae2a660730.jpeg"
]
}
Xóa hoặc sửa xong nó sẽ ko Binding được nữa. Có cách nào giải quyết ko ad?
khi table cần có 3 trường mới thành khóa chính thì phải làm sao để tìm ra đúng mục cần xóa sửa vậy a
Làm sao để có gợi ý code trong sql vậy ạ. Em mới cài bản 2019 ạ.
Với cả làm sao khi code trong VS tự xuống hàng khi đến biên v ạ.
Em cám ơn ạ.
lúc dùng DataSourceUpdateMode thì khi thực hiện 1 thap tác không thể binding được thì sao h